array, list, vector 등의 객체 에서 최대값, 최소값을 구하는 일이 생각 보다 많다.
다만 최대값, 최소값을 구하기 위해서 코드를 얼마나 작성하느냐의 차이가 있다.
C++에서는 이 기능을 #include <algorithm>에서 제공한다.
max_element()나 min_element()는 둘다 모든 요소에 접근을 해야 하기 때문에, 모든 STL 컨테이너에 대해서 선형으로 동작한다. 즉 시간 복잡도가 O(n)이다.
template <class ForwardIterator>
ForwardIterator max_element (ForwardIterator first, ForwardIterator last);
template <class ForwardIterator, class Compare>
ForwardIterator max_element (ForwardIterator first, ForwardIterator last, Compare comp);
template <class ForwardIterator>
ForwardIterator min_element (ForwardIterator first, ForwardIterator last);
template <class ForwardIterator, class Compare>
ForwardIterator min_element (ForwardIterator first, ForwardIterator last, Compare comp);
max_element()나 min_element()는 iterator(반복자)를 return한다.
따라서 max_element()와 min_element() 사용의 가장 큰 장점은 iterator사용이 가능하다는 것이다.
#include <iostream>
#include <algorithm>
#include <vector>
#include <list>
int main() {
int test_arr[] = { 2, 4, 3, 6, 1, 7 };
std::vector<int> test_vec = { 2, 4, 3, 6, 1, 7 };
std::list<int> test_list = { 2, 4, 3, 6, 1, 7 };
std::cout << "min array: " << *std::min_element(test_arr, test_arr + 6) << std::endl;
std::cout << "max array: " << *std::max_element(test_arr, test_arr + 6) << std::endl;
std::cout << "min vector: " << *std::min_element(test_vec.begin(), test_vec.end()) << std::endl;
std::cout << "max vector: " << *std::max_element(test_vec.begin(), test_vec.end()) << std::endl;
std::cout << "min list: " << *std::min_element(test_list.begin(), test_list.end()) << std::endl;
std::cout << "max list: " << *std::max_element(test_list.begin(), test_list.end()) << std::endl;
return 0;
}
추가적으로 max_element나 min_element나 마지막으로 comp라는 이름의 매개변수를 가진다는 것을 알 수 있다.
- 사용조건
- 앞의 두 요소의 범위를 인수로 받아들이고, bool로 반환하는 함수를 사용가능하다.
- 이 bool타입 함수는 인수를 수정하면 안된다.
- 함수 포인터 이거나 함수 객체를 매개변수로 사용 가능하다.
- 첫번째 인수가, 두번째 인수보다 작은지 판단하는 알고리즘으로 작성해야한다.
comp를 제공하는 함수는 위의 기본적인 틀에 따라, 참과 거짓을 나타내는 custom된 함수를 이용 할 수 있다.
- comp 활용
#include <iostream>
#include <algorithm>
bool test(int i, int j) { return(std::abs(i) < std::abs(j)); }
int main() {
int buf[] = { 1, 5, 3, 4, 1, 2, -14 };
std::cout << "min test: " << *std::min_element(buf, buf + 7, test) << std::endl;
std::cout << "max test: " << *std::max_element(buf, buf + 7, test) << std::endl;
return 0;
}
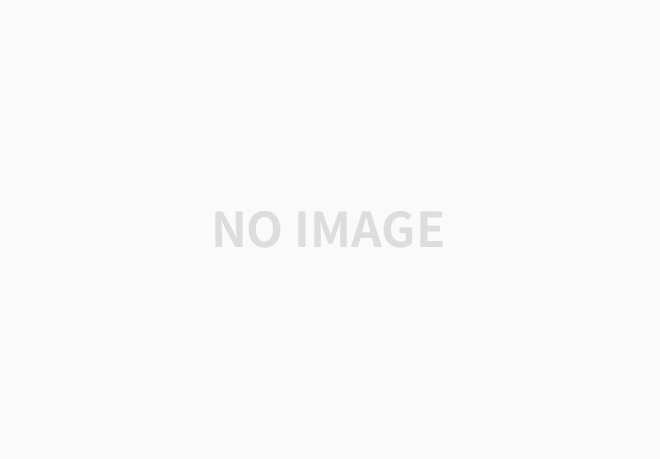
std::abs()는 절대값을 구하는 함수이다. 결국 위의 예제코드에서 min_element와 max_element의 동작은 각각 절대값이 가장 작은 요소와 절대값이 가장 큰 요소를 구하는 함수로서 동작하게 된다.
#출처 & 참고
'Programming > C++' 카테고리의 다른 글
c++ 10진법 진법 변환(string) (0) | 2021.01.17 |
---|---|
C++ and 비트연산을 이용한 홀수, 짝수 판별 (0) | 2020.09.21 |
C++ 입출력 속도 증가(알고리즘 대회) (0) | 2020.09.20 |
C++ string find를 이용한 찾은 문자열 위치 저장 (0) | 2020.07.22 |
C++ vector<vector<int> > 2차원 벡터의 크기 (0) | 2020.07.19 |